forked from lix-project/hydra
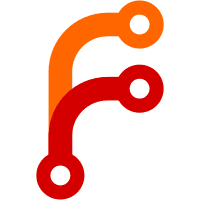
fix build after such commits as df552ff53e68dff8ca360adbdbea214ece1d08ee and e862833ec662c1bffbe31b9a229147de391e801a
53 lines
1.4 KiB
C++
53 lines
1.4 KiB
C++
#pragma once
|
|
|
|
#include <map>
|
|
|
|
#include "util.hh"
|
|
|
|
struct HydraConfig
|
|
{
|
|
std::map<std::string, std::string> options;
|
|
|
|
HydraConfig()
|
|
{
|
|
using namespace nix;
|
|
|
|
/* Read hydra.conf. */
|
|
auto hydraConfigFile = getEnv("HYDRA_CONFIG");
|
|
if (hydraConfigFile && pathExists(*hydraConfigFile)) {
|
|
|
|
for (auto line : tokenizeString<Strings>(readFile(*hydraConfigFile), "\n")) {
|
|
line = trim(std::string(line, 0, line.find('#')));
|
|
|
|
auto eq = line.find('=');
|
|
if (eq == std::string::npos) continue;
|
|
|
|
auto key = trim(std::string(line, 0, eq));
|
|
auto value = trim(std::string(line, eq + 1));
|
|
|
|
if (key == "") continue;
|
|
|
|
options[key] = value;
|
|
}
|
|
}
|
|
}
|
|
|
|
std::string getStrOption(const std::string & key, const std::string & def = "")
|
|
{
|
|
auto i = options.find(key);
|
|
return i == options.end() ? def : i->second;
|
|
}
|
|
|
|
uint64_t getIntOption(const std::string & key, uint64_t def = 0)
|
|
{
|
|
auto i = options.find(key);
|
|
return i == options.end() ? def : std::stoll(i->second);
|
|
}
|
|
|
|
bool getBoolOption(const std::string & key, bool def = false)
|
|
{
|
|
auto i = options.find(key);
|
|
return i == options.end() ? def : (i->second == "true" || i->second == "1");
|
|
}
|
|
};
|