forked from lix-project/lix
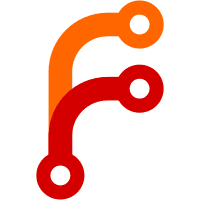
Add a new `init()` method to the `Store` class that is supposed to handle all the effectful initialisation needed to set-up the store. The constructor should remain side-effect free and just initialize the c++ data structure. The goal behind that is that we can create “dummy” instances of each store to query static properties about it (the parameters it accepts for example)
88 lines
2.4 KiB
C++
88 lines
2.4 KiB
C++
#include "store-api.hh"
|
|
#include "remote-store.hh"
|
|
#include "remote-fs-accessor.hh"
|
|
#include "archive.hh"
|
|
#include "worker-protocol.hh"
|
|
#include "pool.hh"
|
|
#include "ssh.hh"
|
|
|
|
namespace nix {
|
|
|
|
class SSHStore : public RemoteStore
|
|
{
|
|
public:
|
|
|
|
const Setting<Path> sshKey{(Store*) this, "", "ssh-key", "path to an SSH private key"};
|
|
const Setting<bool> compress{(Store*) this, false, "compress", "whether to compress the connection"};
|
|
const Setting<Path> remoteProgram{(Store*) this, "nix-daemon", "remote-program", "path to the nix-daemon executable on the remote system"};
|
|
const Setting<std::string> remoteStore{(Store*) this, "", "remote-store", "URI of the store on the remote system"};
|
|
|
|
SSHStore(
|
|
const Params & params)
|
|
: SSHStore("dummy", params)
|
|
{
|
|
}
|
|
|
|
SSHStore(const std::string & host, const Params & params)
|
|
: Store(params)
|
|
, RemoteStore(params)
|
|
, host(host)
|
|
, master(
|
|
host,
|
|
sshKey,
|
|
// Use SSH master only if using more than 1 connection.
|
|
connections->capacity() > 1,
|
|
compress)
|
|
{
|
|
}
|
|
|
|
static std::vector<std::string> uriPrefixes() { return {"ssh-ng"}; }
|
|
|
|
std::string getUri() override
|
|
{
|
|
return uriPrefixes()[0] + "://" + host;
|
|
}
|
|
|
|
bool sameMachine() override
|
|
{ return false; }
|
|
|
|
private:
|
|
|
|
struct Connection : RemoteStore::Connection
|
|
{
|
|
std::unique_ptr<SSHMaster::Connection> sshConn;
|
|
};
|
|
|
|
ref<RemoteStore::Connection> openConnection() override;
|
|
|
|
std::string host;
|
|
|
|
SSHMaster master;
|
|
|
|
void setOptions(RemoteStore::Connection & conn) override
|
|
{
|
|
/* TODO Add a way to explicitly ask for some options to be
|
|
forwarded. One option: A way to query the daemon for its
|
|
settings, and then a series of params to SSHStore like
|
|
forward-cores or forward-overridden-cores that only
|
|
override the requested settings.
|
|
*/
|
|
};
|
|
};
|
|
|
|
ref<RemoteStore::Connection> SSHStore::openConnection()
|
|
{
|
|
auto conn = make_ref<Connection>();
|
|
conn->sshConn = master.startCommand(
|
|
fmt("%s --stdio", remoteProgram)
|
|
+ (remoteStore.get() == "" ? "" : " --store " + shellEscape(remoteStore.get())));
|
|
conn->to = FdSink(conn->sshConn->in.get());
|
|
conn->from = FdSource(conn->sshConn->out.get());
|
|
initConnection(*conn);
|
|
return conn;
|
|
}
|
|
|
|
static RegisterStoreImplementation<SSHStore> regStore;
|
|
|
|
}
|