forked from lix-project/lix
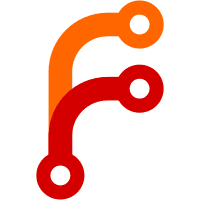
Use `set -u` and `set -o pipefail` to catch accidental mistakes and failures more strongly. - `set -u` catches the use of undefined variables - `set -o pipefail` catches failures (like `set -e`) earlier in the pipeline. This makes the tests a bit more robust. It is nice to read code not worrying about these spurious success paths (via uncaught) errors undermining the tests. Indeed, I caught some bugs doing this. There are a few tests where we run a command that should fail, and then search its output to make sure the failure message is one that we expect. Before, since the `grep` was the last command in the pipeline the exit code of those failing programs was silently ignored. Now with `set -o pipefail` it won't be, and we have to do something so the expected failure doesn't accidentally fail the test. To do that we use `expect` and a new `expectStderr` to check for the exact failing exit code. See the comments on each for why. `grep -q` is replaced with `grepQuiet`, see the comments on that function for why. `grep -v` when we just want the exit code is replaced with `grepInverse, see the comments on that function for why. `grep -q -v` together is, surprise surprise, replaced with `grepQuietInverse`, which is both combined. Co-authored-by: Robert Hensing <roberth@users.noreply.github.com>
62 lines
2.7 KiB
Bash
62 lines
2.7 KiB
Bash
source common.sh
|
||
|
||
clearStore
|
||
|
||
rm -rf $TEST_HOME
|
||
|
||
tarroot=$TEST_ROOT/tarball
|
||
rm -rf $tarroot
|
||
mkdir -p $tarroot
|
||
cp dependencies.nix $tarroot/default.nix
|
||
cp config.nix dependencies.builder*.sh $tarroot/
|
||
|
||
hash=$(nix hash path $tarroot)
|
||
|
||
test_tarball() {
|
||
local ext="$1"
|
||
local compressor="$2"
|
||
|
||
tarball=$TEST_ROOT/tarball.tar$ext
|
||
(cd $TEST_ROOT && tar cf - tarball) | $compressor > $tarball
|
||
|
||
nix-env -f file://$tarball -qa --out-path | grepQuiet dependencies
|
||
|
||
nix-build -o $TEST_ROOT/result file://$tarball
|
||
|
||
nix-build -o $TEST_ROOT/result '<foo>' -I foo=file://$tarball
|
||
|
||
nix-build -o $TEST_ROOT/result -E "import (fetchTarball file://$tarball)"
|
||
# Do not re-fetch paths already present
|
||
nix-build -o $TEST_ROOT/result -E "import (fetchTarball { url = file:///does-not-exist/must-remain-unused/$tarball; sha256 = \"$hash\"; })"
|
||
|
||
nix-build -o $TEST_ROOT/result -E "import (fetchTree file://$tarball)"
|
||
nix-build -o $TEST_ROOT/result -E "import (fetchTree { type = \"tarball\"; url = file://$tarball; })"
|
||
nix-build -o $TEST_ROOT/result -E "import (fetchTree { type = \"tarball\"; url = file://$tarball; narHash = \"$hash\"; })"
|
||
# Do not re-fetch paths already present
|
||
nix-build -o $TEST_ROOT/result -E "import (fetchTree { type = \"tarball\"; url = file:///does-not-exist/must-remain-unused/$tarball; narHash = \"$hash\"; })"
|
||
expectStderr 102 nix-build -o $TEST_ROOT/result -E "import (fetchTree { type = \"tarball\"; url = file://$tarball; narHash = \"sha256-xdKv2pq/IiwLSnBBJXW8hNowI4MrdZfW+SYqDQs7Tzc=\"; })" | grep 'NAR hash mismatch in input'
|
||
|
||
nix-instantiate --strict --eval -E "!((import (fetchTree { type = \"tarball\"; url = file://$tarball; narHash = \"$hash\"; })) ? submodules)" >&2
|
||
nix-instantiate --strict --eval -E "!((import (fetchTree { type = \"tarball\"; url = file://$tarball; narHash = \"$hash\"; })) ? submodules)" 2>&1 | grep 'true'
|
||
|
||
nix-instantiate --eval -E '1 + 2' -I fnord=file://no-such-tarball.tar$ext
|
||
nix-instantiate --eval -E 'with <fnord/xyzzy>; 1 + 2' -I fnord=file://no-such-tarball$ext
|
||
(! nix-instantiate --eval -E '<fnord/xyzzy> 1' -I fnord=file://no-such-tarball$ext)
|
||
|
||
nix-instantiate --eval -E '<fnord/config.nix>' -I fnord=file://no-such-tarball$ext -I fnord=.
|
||
|
||
# Ensure that the `name` attribute isn’t accepted as that would mess
|
||
# with the content-addressing
|
||
(! nix-instantiate --eval -E "fetchTree { type = \"tarball\"; url = file://$tarball; narHash = \"$hash\"; name = \"foo\"; }")
|
||
|
||
}
|
||
|
||
test_tarball '' cat
|
||
test_tarball .xz xz
|
||
test_tarball .gz gzip
|
||
|
||
rm -rf $TEST_ROOT/tmp
|
||
mkdir -p $TEST_ROOT/tmp
|
||
(! TMPDIR=$TEST_ROOT/tmp XDG_RUNTIME_DIR=$TEST_ROOT/tmp nix-env -f file://$(pwd)/bad.tar.xz -qa --out-path)
|
||
(! [ -e $TEST_ROOT/tmp/bad ])
|