forked from lix-project/hydra
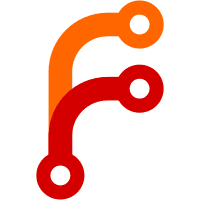
In your hydra config, you can add an arbitrary number of <s3config> sections, with the following options: * name (required): Bucket name * jobs (required): A regex to match job names (in project:jobset:job format) that should be backed up to this bucket * compression_type: bzip2 (default), xz, or none * prefix: String to prepend to all hydra-created s3 keys (if this is meant to represent a directory, you should include the trailing slash, e.g. "cache/"). Default "". After each build with an output (i.e. successful or failed-with-output builds), the output path and its closure are uploaded to the bucket as .nar files, with corresponding .narinfos to enable use as a binary cache. This plugin requires that s3 credentials be available. It uses Net::Amazon::S3, which as of this commit the nixpkgs version can retrieve s3 credentials from the AWS_ACCESS_KEY_ID and AWS_SECRET_ACCESS_KEY environment variables, or from ec2 instance metadata when using an IAM role. This commit also adds a hydra-s3-backup-collect-garbage program, which uses hydra's gc roots directory to determine which paths are live, and then deletes all files except nix-cache-info and any .nar or .narinfo files corresponding to live paths. hydra-s3-backup-collect-garbage respects the prefix configuration option, so it won't delete anything outside of the hierarchy you give it, and it has the same credential requirements as the plugin. Probably a timer unit running the garbage collection periodically should be added to hydra-module.nix Note that two of the added tests fail, due to a bug in the interaction between Net::Amazon::S3 and fake-s3. Those behaviors work against real s3 though, so I'm committing this even with the broken tests. Signed-off-by: Shea Levy <shea@shealevy.com>
89 lines
2.8 KiB
Perl
89 lines
2.8 KiB
Perl
package Setup;
|
|
|
|
use strict;
|
|
use Exporter;
|
|
use Hydra::Helper::Nix;
|
|
use Hydra::Model::DB;
|
|
use Hydra::Helper::AddBuilds;
|
|
use Cwd;
|
|
|
|
our @ISA = qw(Exporter);
|
|
our @EXPORT = qw(hydra_setup nrBuildsForJobset queuedBuildsForJobset nrQueuedBuildsForJobset createBaseJobset createJobsetWithOneInput evalSucceeds runBuild updateRepository);
|
|
|
|
sub hydra_setup {
|
|
my ($db) = @_;
|
|
$db->resultset('Users')->create({ username => "root", emailaddress => 'root@invalid.org', password => '' });
|
|
}
|
|
|
|
sub nrBuildsForJobset {
|
|
my ($jobset) = @_;
|
|
return $jobset->builds->search({},{})->count ;
|
|
}
|
|
|
|
sub queuedBuildsForJobset {
|
|
my ($jobset) = @_;
|
|
return $jobset->builds->search({finished => 0});
|
|
}
|
|
|
|
sub nrQueuedBuildsForJobset {
|
|
my ($jobset) = @_;
|
|
return queuedBuildsForJobset($jobset)->count ;
|
|
}
|
|
|
|
sub createBaseJobset {
|
|
my ($jobsetName, $nixexprpath) = @_;
|
|
|
|
my $db = Hydra::Model::DB->new;
|
|
my $project = $db->resultset('Projects')->update_or_create({name => "tests", displayname => "", owner => "root"});
|
|
my $jobset = $project->jobsets->create({name => $jobsetName, nixexprinput => "jobs", nixexprpath => $nixexprpath, emailoverride => ""});
|
|
|
|
my $jobsetinput;
|
|
my $jobsetinputals;
|
|
|
|
$jobsetinput = $jobset->jobsetinputs->create({name => "jobs", type => "path"});
|
|
$jobsetinputals = $jobsetinput->jobsetinputalts->create({altnr => 0, value => getcwd."/jobs"});
|
|
|
|
return $jobset;
|
|
}
|
|
|
|
sub createJobsetWithOneInput {
|
|
my ($jobsetName, $nixexprpath, $name, $type, $uri) = @_;
|
|
my $jobset = createBaseJobset($jobsetName, $nixexprpath);
|
|
|
|
my $jobsetinput;
|
|
my $jobsetinputals;
|
|
|
|
$jobsetinput = $jobset->jobsetinputs->create({name => $name, type => $type});
|
|
$jobsetinputals = $jobsetinput->jobsetinputalts->create({altnr => 0, value => $uri});
|
|
|
|
return $jobset;
|
|
}
|
|
|
|
sub evalSucceeds {
|
|
my ($jobset) = @_;
|
|
my ($res, $stdout, $stderr) = captureStdoutStderr(60, ("hydra-evaluator", $jobset->project->name, $jobset->name));
|
|
chomp $stdout; chomp $stderr;
|
|
print STDERR "Evaluation errors for jobset ".$jobset->project->name.":".$jobset->name.": \n".$jobset->errormsg."\n" if $jobset->errormsg;
|
|
print STDERR "STDOUT: $stdout\n" if $stdout ne "";
|
|
print STDERR "STDERR: $stderr\n" if $stderr ne "";
|
|
return !$res;
|
|
}
|
|
|
|
sub runBuild {
|
|
my ($build) = @_;
|
|
my ($res, $stdout, $stderr) = captureStdoutStderr(60, ("hydra-build", $build->id));
|
|
print "STDERR: $stderr" if $stderr ne "";
|
|
return !$res;
|
|
}
|
|
|
|
sub updateRepository {
|
|
my ($scm, $update) = @_;
|
|
my ($res, $stdout, $stderr) = captureStdoutStderr(60, ($update, $scm));
|
|
die "unexpected update error with $scm: $stderr\n" if $res;
|
|
my ($message, $loop, $status) = $stdout =~ m/::(.*) -- (.*) -- (.*)::/;
|
|
print STDOUT "Update $scm repository: $message\n";
|
|
return ($loop eq "continue", $status eq "updated");
|
|
}
|
|
|
|
1;
|