forked from lix-project/hydra
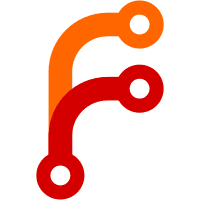
This rewrites the top-level loop of hydra-evaluator in C++. The Perl stuff is moved into hydra-eval-jobset. (Rewriting the entire evaluator would be nice but is a bit too much work.) The new version has some advantages: * It can run multiple jobset evaluations in parallel. * It uses PostgreSQL notifications so it doesn't have to poll the database. So if a jobset is triggered via the web interface or from a GitHub / Bitbucket webhook, evaluation of the jobset will start almost instantaneously (assuming the evaluator is not at its concurrency limit). * It imposes a timeout on evaluations. So if e.g. hydra-eval-jobset hangs connecting to a Mercurial server, it will eventually be killed.
50 lines
2 KiB
Perl
50 lines
2 KiB
Perl
use strict;
|
|
use File::Basename;
|
|
use Hydra::Model::DB;
|
|
use Hydra::Helper::Nix;
|
|
use Nix::Store;
|
|
use Cwd;
|
|
|
|
my $db = Hydra::Model::DB->new;
|
|
|
|
use Test::Simple tests => 6;
|
|
|
|
$db->resultset('Users')->create({ username => "root", emailaddress => 'root@invalid.org', password => '' });
|
|
|
|
$db->resultset('Projects')->create({name => "tests", displayname => "", owner => "root"});
|
|
my $project = $db->resultset('Projects')->update_or_create({name => "tests", displayname => "", owner => "root"});
|
|
my $jobset = $project->jobsets->create({name => "basic", nixexprinput => "jobs", nixexprpath => "default.nix", emailoverride => ""});
|
|
|
|
my $jobsetinput;
|
|
|
|
$jobsetinput = $jobset->jobsetinputs->create({name => "jobs", type => "path"});
|
|
$jobsetinput->jobsetinputalts->create({altnr => 0, value => getcwd . "/jobs"});
|
|
system("hydra-eval-jobset " . $jobset->project->name . " " . $jobset->name);
|
|
|
|
my $successful_hash;
|
|
foreach my $build ($jobset->builds->search({finished => 0})) {
|
|
system("hydra-build " . $build->id);
|
|
my @outputs = $build->buildoutputs->all;
|
|
my $hash = substr basename($outputs[0]->path), 0, 32;
|
|
if ($build->job->name eq "job") {
|
|
ok(-e "/tmp/s3/hydra/$hash.nar", "The nar of a successful matched build is uploaded");
|
|
ok(-e "/tmp/s3/hydra/$hash.narinfo", "The narinfo of a successful matched build is uploaded");
|
|
$successful_hash = $hash;
|
|
}
|
|
}
|
|
|
|
system("hydra-s3-backup-collect-garbage");
|
|
ok(-e "/tmp/s3/hydra/$successful_hash.nar", "The nar of a build that's a root is not removed by gc");
|
|
ok(-e "/tmp/s3/hydra/$successful_hash.narinfo", "The narinfo of a build that's a root is not removed by gc");
|
|
|
|
my $gcRootsDir = getGCRootsDir;
|
|
opendir DIR, $gcRootsDir or die;
|
|
while(readdir DIR) {
|
|
next if $_ eq "." or $_ eq "..";
|
|
unlink "$gcRootsDir/$_";
|
|
}
|
|
closedir DIR;
|
|
system("hydra-s3-backup-collect-garbage");
|
|
ok(not -e "/tmp/s3/hydra/$successful_hash.nar", "The nar of a build that's not a root is removed by gc");
|
|
ok(not -e "/tmp/s3/hydra/$successful_hash.narinfo", "The narinfo of a build that's not a root is removed by gc");
|