forked from lix-project/lix
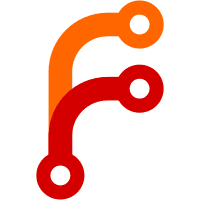
Today, with the tests inside a `tests` intermingled with the corresponding library's source code, we have a few problems: - We have to be careful that wildcards don't end up with tests being built as part of Nix proper, or test headers being installed as part of Nix proper. - Tests in libraries but not executables is not right: - It means each executable runs the previous unit tests again, because it needs the libraries. - It doesn't work right on Windows, which doesn't want you to load a DLL just for the side global variable . It could be made to work with the dlopen equivalent, but that's gross! This reorg solves these problems. There is a remaining problem which is that sibbling headers (like `hash.hh` the test header vs `hash.hh` the main `libnixutil` header) end up shadowing each other. This PR doesn't solve that. That is left as future work for a future PR. Co-authored-by: Valentin Gagarin <valentin.gagarin@tweag.io> (cherry picked from commit 91b6833686a6a6d9eac7f3f66393ec89ef1d3b57) (cherry picked from commit a61e42adb528b3d40ce43e07c79368d779a8b624)
69 lines
2 KiB
C++
69 lines
2 KiB
C++
#include <nlohmann/json.hpp>
|
|
#include <gtest/gtest.h>
|
|
#include <rapidcheck/gtest.h>
|
|
|
|
#include "tests/derived-path.hh"
|
|
#include "tests/libexpr.hh"
|
|
|
|
namespace nix {
|
|
|
|
// Testing of trivial expressions
|
|
class DerivedPathExpressionTest : public LibExprTest {};
|
|
|
|
// FIXME: `RC_GTEST_FIXTURE_PROP` isn't calling `SetUpTestSuite` because it is
|
|
// no a real fixture.
|
|
//
|
|
// See https://github.com/emil-e/rapidcheck/blob/master/doc/gtest.md#rc_gtest_fixture_propfixture-name-args
|
|
TEST_F(DerivedPathExpressionTest, force_init)
|
|
{
|
|
}
|
|
|
|
#ifndef COVERAGE
|
|
|
|
RC_GTEST_FIXTURE_PROP(
|
|
DerivedPathExpressionTest,
|
|
prop_opaque_path_round_trip,
|
|
(const SingleDerivedPath::Opaque & o))
|
|
{
|
|
auto * v = state.allocValue();
|
|
state.mkStorePathString(o.path, *v);
|
|
auto d = state.coerceToSingleDerivedPath(noPos, *v, "");
|
|
RC_ASSERT(SingleDerivedPath { o } == d);
|
|
}
|
|
|
|
// TODO use DerivedPath::Built for parameter once it supports a single output
|
|
// path only.
|
|
|
|
RC_GTEST_FIXTURE_PROP(
|
|
DerivedPathExpressionTest,
|
|
prop_derived_path_built_placeholder_round_trip,
|
|
(const SingleDerivedPath::Built & b))
|
|
{
|
|
/**
|
|
* We set these in tests rather than the regular globals so we don't have
|
|
* to worry about race conditions if the tests run concurrently.
|
|
*/
|
|
ExperimentalFeatureSettings mockXpSettings;
|
|
mockXpSettings.set("experimental-features", "ca-derivations");
|
|
|
|
auto * v = state.allocValue();
|
|
state.mkOutputString(*v, b, std::nullopt, mockXpSettings);
|
|
auto [d, _] = state.coerceToSingleDerivedPathUnchecked(noPos, *v, "");
|
|
RC_ASSERT(SingleDerivedPath { b } == d);
|
|
}
|
|
|
|
RC_GTEST_FIXTURE_PROP(
|
|
DerivedPathExpressionTest,
|
|
prop_derived_path_built_out_path_round_trip,
|
|
(const SingleDerivedPath::Built & b, const StorePath & outPath))
|
|
{
|
|
auto * v = state.allocValue();
|
|
state.mkOutputString(*v, b, outPath);
|
|
auto [d, _] = state.coerceToSingleDerivedPathUnchecked(noPos, *v, "");
|
|
RC_ASSERT(SingleDerivedPath { b } == d);
|
|
}
|
|
|
|
#endif
|
|
|
|
} /* namespace nix */
|