forked from lix-project/lix
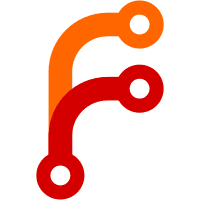
The binary cache store can now use HTTP/2 to do lookups. This is much more efficient than HTTP/1.1 due to multiplexing: we can issue many requests in parallel over a single TCP connection. Thus it's no longer necessary to use a bunch of concurrent TCP connections (25 by default). For example, downloading 802 .narinfo files from https://cache.nixos.org/, using a single TCP connection, takes 11.8s with HTTP/1.1, but only 0.61s with HTTP/2. This did require a fairly substantial rewrite of the Downloader class to use the curl multi interface, because otherwise curl wouldn't be able to do multiplexing for us. As a bonus, we get connection reuse even with HTTP/1.1. All downloads are now handled by a single worker thread. Clients call Downloader::enqueueDownload() to tell the worker thread to start the download, getting a std::future to the result.
68 lines
1.6 KiB
C++
68 lines
1.6 KiB
C++
#include "common-opts.hh"
|
||
#include "shared.hh"
|
||
#include "download.hh"
|
||
#include "util.hh"
|
||
|
||
|
||
namespace nix {
|
||
|
||
|
||
bool parseAutoArgs(Strings::iterator & i,
|
||
const Strings::iterator & argsEnd, std::map<string, string> & res)
|
||
{
|
||
string arg = *i;
|
||
if (arg != "--arg" && arg != "--argstr") return false;
|
||
|
||
UsageError error(format("‘%1%’ requires two arguments") % arg);
|
||
|
||
if (++i == argsEnd) throw error;
|
||
string name = *i;
|
||
if (++i == argsEnd) throw error;
|
||
string value = *i;
|
||
|
||
res[name] = (arg == "--arg" ? 'E' : 'S') + value;
|
||
|
||
return true;
|
||
}
|
||
|
||
|
||
Bindings * evalAutoArgs(EvalState & state, std::map<string, string> & in)
|
||
{
|
||
Bindings * res = state.allocBindings(in.size());
|
||
for (auto & i : in) {
|
||
Value * v = state.allocValue();
|
||
if (i.second[0] == 'E')
|
||
state.mkThunk_(*v, state.parseExprFromString(string(i.second, 1), absPath(".")));
|
||
else
|
||
mkString(*v, string(i.second, 1));
|
||
res->push_back(Attr(state.symbols.create(i.first), v));
|
||
}
|
||
res->sort();
|
||
return res;
|
||
}
|
||
|
||
|
||
bool parseSearchPathArg(Strings::iterator & i,
|
||
const Strings::iterator & argsEnd, Strings & searchPath)
|
||
{
|
||
if (*i != "-I") return false;
|
||
if (++i == argsEnd) throw UsageError("‘-I’ requires an argument");
|
||
searchPath.push_back(*i);
|
||
return true;
|
||
}
|
||
|
||
|
||
Path lookupFileArg(EvalState & state, string s)
|
||
{
|
||
if (isUri(s))
|
||
return getDownloader()->downloadCached(state.store, s, true);
|
||
else if (s.size() > 2 && s.at(0) == '<' && s.at(s.size() - 1) == '>') {
|
||
Path p = s.substr(1, s.size() - 2);
|
||
return state.findFile(p);
|
||
} else
|
||
return absPath(s);
|
||
}
|
||
|
||
|
||
}
|