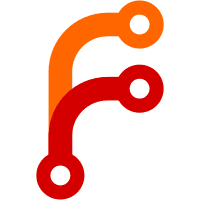
Today, with the tests inside a `tests` intermingled with the corresponding library's source code, we have a few problems: - We have to be careful that wildcards don't end up with tests being built as part of Nix proper, or test headers being installed as part of Nix proper. - Tests in libraries but not executables is not right: - It means each executable runs the previous unit tests again, because it needs the libraries. - It doesn't work right on Windows, which doesn't want you to load a DLL just for the side global variable . It could be made to work with the dlopen equivalent, but that's gross! This reorg solves these problems. There is a remaining problem which is that sibbling headers (like `hash.hh` the test header vs `hash.hh` the main `libnixutil` header) end up shadowing each other. This PR doesn't solve that. That is left as future work for a future PR. Co-authored-by: Valentin Gagarin <valentin.gagarin@tweag.io> (cherry picked from commit 91b6833686a6a6d9eac7f3f66393ec89ef1d3b57) (cherry picked from commit a61e42adb528b3d40ce43e07c79368d779a8b624)
69 lines
1.8 KiB
C++
69 lines
1.8 KiB
C++
#include "tests/libexpr.hh"
|
|
#include "value-to-json.hh"
|
|
|
|
namespace nix {
|
|
// Testing the conversion to JSON
|
|
|
|
class JSONValueTest : public LibExprTest {
|
|
protected:
|
|
std::string getJSONValue(Value& value) {
|
|
std::stringstream ss;
|
|
NixStringContext ps;
|
|
printValueAsJSON(state, true, value, noPos, ss, ps);
|
|
return ss.str();
|
|
}
|
|
};
|
|
|
|
TEST_F(JSONValueTest, null) {
|
|
Value v;
|
|
v.mkNull();
|
|
ASSERT_EQ(getJSONValue(v), "null");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, BoolFalse) {
|
|
Value v;
|
|
v.mkBool(false);
|
|
ASSERT_EQ(getJSONValue(v),"false");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, BoolTrue) {
|
|
Value v;
|
|
v.mkBool(true);
|
|
ASSERT_EQ(getJSONValue(v), "true");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, IntPositive) {
|
|
Value v;
|
|
v.mkInt(100);
|
|
ASSERT_EQ(getJSONValue(v), "100");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, IntNegative) {
|
|
Value v;
|
|
v.mkInt(-100);
|
|
ASSERT_EQ(getJSONValue(v), "-100");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, String) {
|
|
Value v;
|
|
v.mkString("test");
|
|
ASSERT_EQ(getJSONValue(v), "\"test\"");
|
|
}
|
|
|
|
TEST_F(JSONValueTest, StringQuotes) {
|
|
Value v;
|
|
|
|
v.mkString("test\"");
|
|
ASSERT_EQ(getJSONValue(v), "\"test\\\"\"");
|
|
}
|
|
|
|
// The dummy store doesn't support writing files. Fails with this exception message:
|
|
// C++ exception with description "error: operation 'addToStoreFromDump' is
|
|
// not supported by store 'dummy'" thrown in the test body.
|
|
TEST_F(JSONValueTest, DISABLED_Path) {
|
|
Value v;
|
|
v.mkPath("test");
|
|
ASSERT_EQ(getJSONValue(v), "\"/nix/store/g1w7hy3qg1w7hy3qg1w7hy3qg1w7hy3q-x\"");
|
|
}
|
|
} /* namespace nix */
|