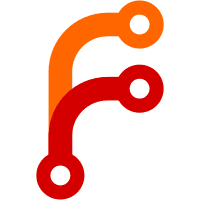
Extend `FSAccessor::readFile` to allow not checking that the path is a valid one, and rewrite `readInvalidDerivation` using this extended `readFile`. Several places in the code use `readInvalidDerivation`, either because they need to read a derivation that has been written in the store but not registered yet, or more generally to prevent a deadlock because `readDerivation` tries to lock the state, so can't be called from a place where the lock is already held. However, `readInvalidDerivation` implicitely assumes that the store is a `LocalFSStore`, which isn't always the case. The concrete motivation for this is that it's required for `nix copy --from someBinaryCache` to work, which is tremendously useful for the tests.
41 lines
1 KiB
C++
41 lines
1 KiB
C++
#pragma once
|
|
|
|
#include "types.hh"
|
|
|
|
namespace nix {
|
|
|
|
/* An abstract class for accessing a filesystem-like structure, such
|
|
as a (possibly remote) Nix store or the contents of a NAR file. */
|
|
class FSAccessor
|
|
{
|
|
public:
|
|
enum Type { tMissing, tRegular, tSymlink, tDirectory };
|
|
|
|
struct Stat
|
|
{
|
|
Type type = tMissing;
|
|
uint64_t fileSize = 0; // regular files only
|
|
bool isExecutable = false; // regular files only
|
|
uint64_t narOffset = 0; // regular files only
|
|
};
|
|
|
|
virtual ~FSAccessor() { }
|
|
|
|
virtual Stat stat(const Path & path) = 0;
|
|
|
|
virtual StringSet readDirectory(const Path & path) = 0;
|
|
|
|
/**
|
|
* Read a file inside the store.
|
|
*
|
|
* If `requireValidPath` is set to `true` (the default), the path must be
|
|
* inside a valid store path, otherwise it just needs to be physically
|
|
* present (but not necessarily properly registered)
|
|
*/
|
|
virtual std::string readFile(const Path & path, bool requireValidPath = true) = 0;
|
|
|
|
virtual std::string readLink(const Path & path) = 0;
|
|
};
|
|
|
|
}
|