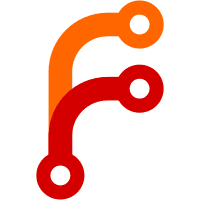
Today, with the tests inside a `tests` intermingled with the corresponding library's source code, we have a few problems: - We have to be careful that wildcards don't end up with tests being built as part of Nix proper, or test headers being installed as part of Nix proper. - Tests in libraries but not executables is not right: - It means each executable runs the previous unit tests again, because it needs the libraries. - It doesn't work right on Windows, which doesn't want you to load a DLL just for the side global variable . It could be made to work with the dlopen equivalent, but that's gross! This reorg solves these problems. There is a remaining problem which is that sibbling headers (like `hash.hh` the test header vs `hash.hh` the main `libnixutil` header) end up shadowing each other. This PR doesn't solve that. That is left as future work for a future PR. Co-authored-by: Valentin Gagarin <valentin.gagarin@tweag.io> (cherry picked from commit 91b6833686a6a6d9eac7f3f66393ec89ef1d3b57) (cherry picked from commit a61e42adb528b3d40ce43e07c79368d779a8b624)
55 lines
1.3 KiB
C++
55 lines
1.3 KiB
C++
#include "chunked-vector.hh"
|
|
|
|
#include <gtest/gtest.h>
|
|
|
|
namespace nix {
|
|
TEST(ChunkedVector, InitEmpty) {
|
|
auto v = ChunkedVector<int, 2>(100);
|
|
ASSERT_EQ(v.size(), 0);
|
|
}
|
|
|
|
TEST(ChunkedVector, GrowsCorrectly) {
|
|
auto v = ChunkedVector<int, 2>(100);
|
|
for (auto i = 1; i < 20; i++) {
|
|
v.add(i);
|
|
ASSERT_EQ(v.size(), i);
|
|
}
|
|
}
|
|
|
|
TEST(ChunkedVector, AddAndGet) {
|
|
auto v = ChunkedVector<int, 2>(100);
|
|
for (auto i = 1; i < 20; i++) {
|
|
auto [i2, idx] = v.add(i);
|
|
auto & i3 = v[idx];
|
|
ASSERT_EQ(i, i2);
|
|
ASSERT_EQ(&i2, &i3);
|
|
}
|
|
}
|
|
|
|
TEST(ChunkedVector, ForEach) {
|
|
auto v = ChunkedVector<int, 2>(100);
|
|
for (auto i = 1; i < 20; i++) {
|
|
v.add(i);
|
|
}
|
|
int count = 0;
|
|
v.forEach([&count](int elt) {
|
|
count++;
|
|
});
|
|
ASSERT_EQ(count, v.size());
|
|
}
|
|
|
|
TEST(ChunkedVector, OverflowOK) {
|
|
// Similar to the AddAndGet, but intentionnally use a small
|
|
// initial ChunkedVector to force it to overflow
|
|
auto v = ChunkedVector<int, 2>(2);
|
|
for (auto i = 1; i < 20; i++) {
|
|
auto [i2, idx] = v.add(i);
|
|
auto & i3 = v[idx];
|
|
ASSERT_EQ(i, i2);
|
|
ASSERT_EQ(&i2, &i3);
|
|
}
|
|
}
|
|
|
|
}
|
|
|