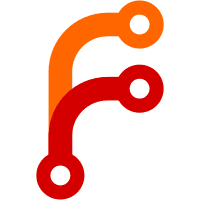
This fetchers copies a plain directory (i.e. not a Git/Mercurial
repository) to the store (or does nothing if the path is already a
store path).
One use case is to pin the 'nixpkgs' flake used to build the current
NixOS system, and prevent it from being garbage-collected, via a
system registry entry like this:
{
"from": {
"id": "nixpkgs",
"type": "indirect"
},
"to": {
"type": "path",
"path": "/nix/store/rralhl3wj4rdwzjn16g7d93mibvlr521-source",
"lastModified": 1585388205,
"rev": "b0c285807d6a9f1b7562ec417c24fa1a30ecc31a"
},
"exact": true
}
Note the fake "lastModified" and "rev" attributes that ensure that the
flake gives the same evaluation results as the corresponding
Git/GitHub inputs.
(cherry picked from commit 12f9379123
)
40 lines
1,019 B
C++
40 lines
1,019 B
C++
#pragma once
|
|
|
|
#include "types.hh"
|
|
|
|
#include <variant>
|
|
|
|
#include <nlohmann/json_fwd.hpp>
|
|
|
|
namespace nix::fetchers {
|
|
|
|
/* Wrap bools to prevent string literals (i.e. 'char *') from being
|
|
cast to a bool in Attr. */
|
|
template<typename T>
|
|
struct Explicit {
|
|
T t;
|
|
};
|
|
|
|
typedef std::variant<std::string, int64_t, Explicit<bool>> Attr;
|
|
typedef std::map<std::string, Attr> Attrs;
|
|
|
|
Attrs jsonToAttrs(const nlohmann::json & json);
|
|
|
|
nlohmann::json attrsToJson(const Attrs & attrs);
|
|
|
|
std::optional<std::string> maybeGetStrAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::string getStrAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::optional<int64_t> maybeGetIntAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
int64_t getIntAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::optional<bool> maybeGetBoolAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
bool getBoolAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::map<std::string, std::string> attrsToQuery(const Attrs & attrs);
|
|
|
|
}
|