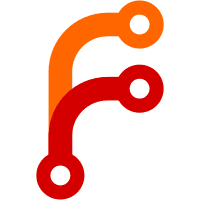
Types converted: - `NixStringContextElem` - `OutputsSpec` - `ExtendedOutputsSpec` - `DerivationOutput` - `DerivationType` Existing ones mostly conforming the pattern cleaned up: - `ContentAddressMethod` - `ContentAddressWithReferences` The `DerivationGoal::derivationType` field had a bogus initialization, now caught, so I made it `std::optional`. I think #8829 can make it non-optional again because it will ensure we always have the derivation when we construct a `DerivationGoal`. See that issue (#7479) for details on the general goal. `git grep 'Raw::Raw'` indicates the two types I didn't yet convert `DerivedPath` and `BuiltPath` (and their `Single` variants) . This is because @roberth and I (can't find issue right now...) plan on reworking them somewhat, so I didn't want to churn them more just yet. Co-authored-by: Eelco Dolstra <edolstra@gmail.com>
66 lines
2.1 KiB
C++
66 lines
2.1 KiB
C++
#include "installable-derived-path.hh"
|
|
#include "derivations.hh"
|
|
|
|
namespace nix {
|
|
|
|
std::string InstallableDerivedPath::what() const
|
|
{
|
|
return derivedPath.to_string(*store);
|
|
}
|
|
|
|
DerivedPathsWithInfo InstallableDerivedPath::toDerivedPaths()
|
|
{
|
|
return {{
|
|
.path = derivedPath,
|
|
.info = make_ref<ExtraPathInfo>(),
|
|
}};
|
|
}
|
|
|
|
std::optional<StorePath> InstallableDerivedPath::getStorePath()
|
|
{
|
|
return derivedPath.getBaseStorePath();
|
|
}
|
|
|
|
InstallableDerivedPath InstallableDerivedPath::parse(
|
|
ref<Store> store,
|
|
std::string_view prefix,
|
|
ExtendedOutputsSpec extendedOutputsSpec)
|
|
{
|
|
auto derivedPath = std::visit(overloaded {
|
|
// If the user did not use ^, we treat the output more
|
|
// liberally: we accept a symlink chain or an actual
|
|
// store path.
|
|
[&](const ExtendedOutputsSpec::Default &) -> DerivedPath {
|
|
auto storePath = store->followLinksToStorePath(prefix);
|
|
// Remove this prior to stabilizing the new CLI.
|
|
if (storePath.isDerivation()) {
|
|
auto oldDerivedPath = DerivedPath::Built {
|
|
.drvPath = makeConstantStorePathRef(storePath),
|
|
.outputs = OutputsSpec::All { },
|
|
};
|
|
warn(
|
|
"The interpretation of store paths arguments ending in `.drv` recently changed. If this command is now failing try again with '%s'",
|
|
oldDerivedPath.to_string(*store));
|
|
};
|
|
return DerivedPath::Opaque {
|
|
.path = std::move(storePath),
|
|
};
|
|
},
|
|
// If the user did use ^, we just do exactly what is written.
|
|
[&](const ExtendedOutputsSpec::Explicit & outputSpec) -> DerivedPath {
|
|
auto drv = make_ref<SingleDerivedPath>(SingleDerivedPath::parse(*store, prefix));
|
|
drvRequireExperiment(*drv);
|
|
return DerivedPath::Built {
|
|
.drvPath = std::move(drv),
|
|
.outputs = outputSpec,
|
|
};
|
|
},
|
|
}, extendedOutputsSpec.raw);
|
|
return InstallableDerivedPath {
|
|
store,
|
|
std::move(derivedPath),
|
|
};
|
|
}
|
|
|
|
}
|