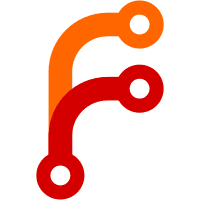
This adds a new store operation 'addMultipleToStore' that reads a number of NARs and ValidPathInfos from a Source, allowing any number of store paths to be copied in a single call. This is much faster on high-latency links when copying a lot of small files, like .drv closures. For example, on a connection with an 50 ms delay: Before: $ nix copy --to 'unix:///tmp/proxy-socket?root=/tmp/dest-chroot' \ /nix/store/90jjw94xiyg5drj70whm9yll6xjj0ca9-hello-2.10.drv \ --derivation --no-check-sigs real 0m57.868s user 0m0.103s sys 0m0.056s After: real 0m0.690s user 0m0.017s sys 0m0.011s
47 lines
1.4 KiB
C++
47 lines
1.4 KiB
C++
#include "path-info.hh"
|
|
#include "worker-protocol.hh"
|
|
|
|
namespace nix {
|
|
|
|
ValidPathInfo ValidPathInfo::read(Source & source, const Store & store, unsigned int format)
|
|
{
|
|
return read(source, store, format, store.parseStorePath(readString(source)));
|
|
}
|
|
|
|
ValidPathInfo ValidPathInfo::read(Source & source, const Store & store, unsigned int format, StorePath && path)
|
|
{
|
|
auto deriver = readString(source);
|
|
auto narHash = Hash::parseAny(readString(source), htSHA256);
|
|
ValidPathInfo info(path, narHash);
|
|
if (deriver != "") info.deriver = store.parseStorePath(deriver);
|
|
info.references = worker_proto::read(store, source, Phantom<StorePathSet> {});
|
|
source >> info.registrationTime >> info.narSize;
|
|
if (format >= 16) {
|
|
source >> info.ultimate;
|
|
info.sigs = readStrings<StringSet>(source);
|
|
info.ca = parseContentAddressOpt(readString(source));
|
|
}
|
|
return info;
|
|
}
|
|
|
|
void ValidPathInfo::write(
|
|
Sink & sink,
|
|
const Store & store,
|
|
unsigned int format,
|
|
bool includePath) const
|
|
{
|
|
if (includePath)
|
|
sink << store.printStorePath(path);
|
|
sink << (deriver ? store.printStorePath(*deriver) : "")
|
|
<< narHash.to_string(Base16, false);
|
|
worker_proto::write(store, sink, references);
|
|
sink << registrationTime << narSize;
|
|
if (format >= 16) {
|
|
sink << ultimate
|
|
<< sigs
|
|
<< renderContentAddress(ca);
|
|
}
|
|
}
|
|
|
|
}
|