forked from lix-project/lix
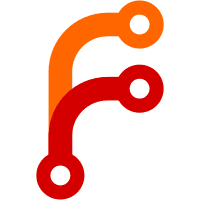
If you do a fetchTree on a Git repository, whether the result contains a revCount attribute should not depend on whether that repository happens to be a shallow clone or not. That would complicate caching a lot and would be semantically messy. So applying fetchTree/fetchGit to a shallow repository is now an error unless you pass the attribute 'shallow = true'. If 'shallow = true', we don't return revCount, even if the repository is not actually shallow. Note that Nix itself is not doing shallow clones at the moment. But it could do so as an optimisation if the user specifies 'shallow = true'. Issue #2988.
38 lines
948 B
C++
38 lines
948 B
C++
#pragma once
|
|
|
|
#include "types.hh"
|
|
|
|
#include <variant>
|
|
|
|
#include <nlohmann/json_fwd.hpp>
|
|
|
|
namespace nix::fetchers {
|
|
|
|
/* Wrap bools to prevent string literals (i.e. 'char *') from being
|
|
cast to a bool in Attr. */
|
|
template<typename T>
|
|
struct Explicit {
|
|
T t;
|
|
};
|
|
|
|
typedef std::variant<std::string, int64_t, Explicit<bool>> Attr;
|
|
typedef std::map<std::string, Attr> Attrs;
|
|
|
|
Attrs jsonToAttrs(const nlohmann::json & json);
|
|
|
|
nlohmann::json attrsToJson(const Attrs & attrs);
|
|
|
|
std::optional<std::string> maybeGetStrAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::string getStrAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::optional<int64_t> maybeGetIntAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
int64_t getIntAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::optional<bool> maybeGetBoolAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
bool getBoolAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
}
|