forked from lix-project/lix
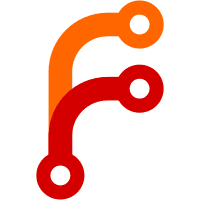
mode. Presumably nix-worker would be setuid to the Nix store user. The worker performs all operations on the Nix store and database, so the caller can be completely unprivileged. This is already much more secure than the old setuid scheme, since the worker doesn't need to do Nix expression evaluation and so on. Most importantly, this means that it doesn't need to access any user files, with all resulting security risks; it only performs pure store operations. Once this works, it is easy to move to a daemon model that forks off a worker for connections established through a Unix domain socket. That would be even more secure.
82 lines
1.5 KiB
C++
82 lines
1.5 KiB
C++
#ifndef __SERIALISE_H
|
|
#define __SERIALISE_H
|
|
|
|
#include "types.hh"
|
|
|
|
|
|
namespace nix {
|
|
|
|
|
|
/* Abstract destination of binary data. */
|
|
struct Sink
|
|
{
|
|
virtual ~Sink() { }
|
|
virtual void operator () (const unsigned char * data, unsigned int len) = 0;
|
|
};
|
|
|
|
|
|
/* Abstract source of binary data. */
|
|
struct Source
|
|
{
|
|
virtual ~Source() { }
|
|
|
|
/* The callee should store exactly *len bytes in the buffer
|
|
pointed to by data. It should block if that much data is not
|
|
yet available, or throw an error if it is not going to be
|
|
available. */
|
|
virtual void operator () (unsigned char * data, unsigned int len) = 0;
|
|
};
|
|
|
|
|
|
/* A sink that writes data to a file descriptor. */
|
|
struct FdSink : Sink
|
|
{
|
|
int fd;
|
|
|
|
FdSink()
|
|
{
|
|
fd = 0;
|
|
}
|
|
|
|
FdSink(int fd)
|
|
{
|
|
this->fd = fd;
|
|
}
|
|
|
|
void operator () (const unsigned char * data, unsigned int len);
|
|
};
|
|
|
|
|
|
/* A source that reads data from a file descriptor. */
|
|
struct FdSource : Source
|
|
{
|
|
int fd;
|
|
|
|
FdSource()
|
|
{
|
|
fd = 0;
|
|
}
|
|
|
|
FdSource(int fd)
|
|
{
|
|
this->fd = fd;
|
|
}
|
|
|
|
void operator () (unsigned char * data, unsigned int len);
|
|
};
|
|
|
|
|
|
void writePadding(unsigned int len, Sink & sink);
|
|
void writeInt(unsigned int n, Sink & sink);
|
|
void writeString(const string & s, Sink & sink);
|
|
|
|
void readPadding(unsigned int len, Source & source);
|
|
unsigned int readInt(Source & source);
|
|
string readString(Source & source);
|
|
|
|
|
|
}
|
|
|
|
|
|
#endif /* !__SERIALISE_H */
|