forked from lix-project/lix
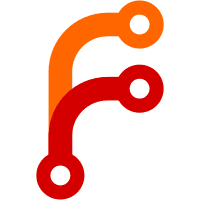
value; this potentially dangerous feature enables better sharing for those paths for which the content is known in advance (e.g., because a content hash is given). * Fast builds: if we can expand all output paths of a derive expression, we don't have to build.
76 lines
1.3 KiB
C++
76 lines
1.3 KiB
C++
#ifndef __FSTATE_H
|
|
#define __FSTATE_H
|
|
|
|
extern "C" {
|
|
#include <aterm2.h>
|
|
}
|
|
|
|
#include "store.hh"
|
|
|
|
|
|
/* Abstract syntax of fstate-expressions. */
|
|
|
|
typedef list<FSId> FSIds;
|
|
|
|
struct SliceElem
|
|
{
|
|
string path;
|
|
FSId id;
|
|
FSIds refs;
|
|
};
|
|
|
|
typedef list<SliceElem> SliceElems;
|
|
|
|
struct Slice
|
|
{
|
|
FSIds roots;
|
|
SliceElems elems;
|
|
};
|
|
|
|
typedef pair<string, FSId> DeriveOutput;
|
|
typedef pair<string, string> StringPair;
|
|
typedef list<DeriveOutput> DeriveOutputs;
|
|
typedef list<StringPair> StringPairs;
|
|
|
|
struct Derive
|
|
{
|
|
DeriveOutputs outputs;
|
|
FSIds inputs;
|
|
string builder;
|
|
string platform;
|
|
StringPairs env;
|
|
};
|
|
|
|
struct FState
|
|
{
|
|
enum { fsSlice, fsDerive } type;
|
|
Slice slice;
|
|
Derive derive;
|
|
};
|
|
|
|
|
|
/* Return a canonical textual representation of an expression. */
|
|
string printTerm(ATerm t);
|
|
|
|
/* Throw an exception with an error message containing the given
|
|
aterm. */
|
|
Error badTerm(const format & f, ATerm t);
|
|
|
|
/* Hash an aterm. */
|
|
Hash hashTerm(ATerm t);
|
|
|
|
/* Read an aterm from disk, given its id. */
|
|
ATerm termFromId(const FSId & id);
|
|
|
|
/* Write an aterm to the Nix store directory, and return its hash. */
|
|
FSId writeTerm(ATerm t, const string & suffix, FSId id = FSId());
|
|
|
|
/* Parse an fstate-expression. */
|
|
FState parseFState(ATerm t);
|
|
|
|
/* Parse an fstate-expression. */
|
|
ATerm unparseFState(const FState & fs);
|
|
|
|
|
|
#endif /* !__FSTATE_H */
|